반응형
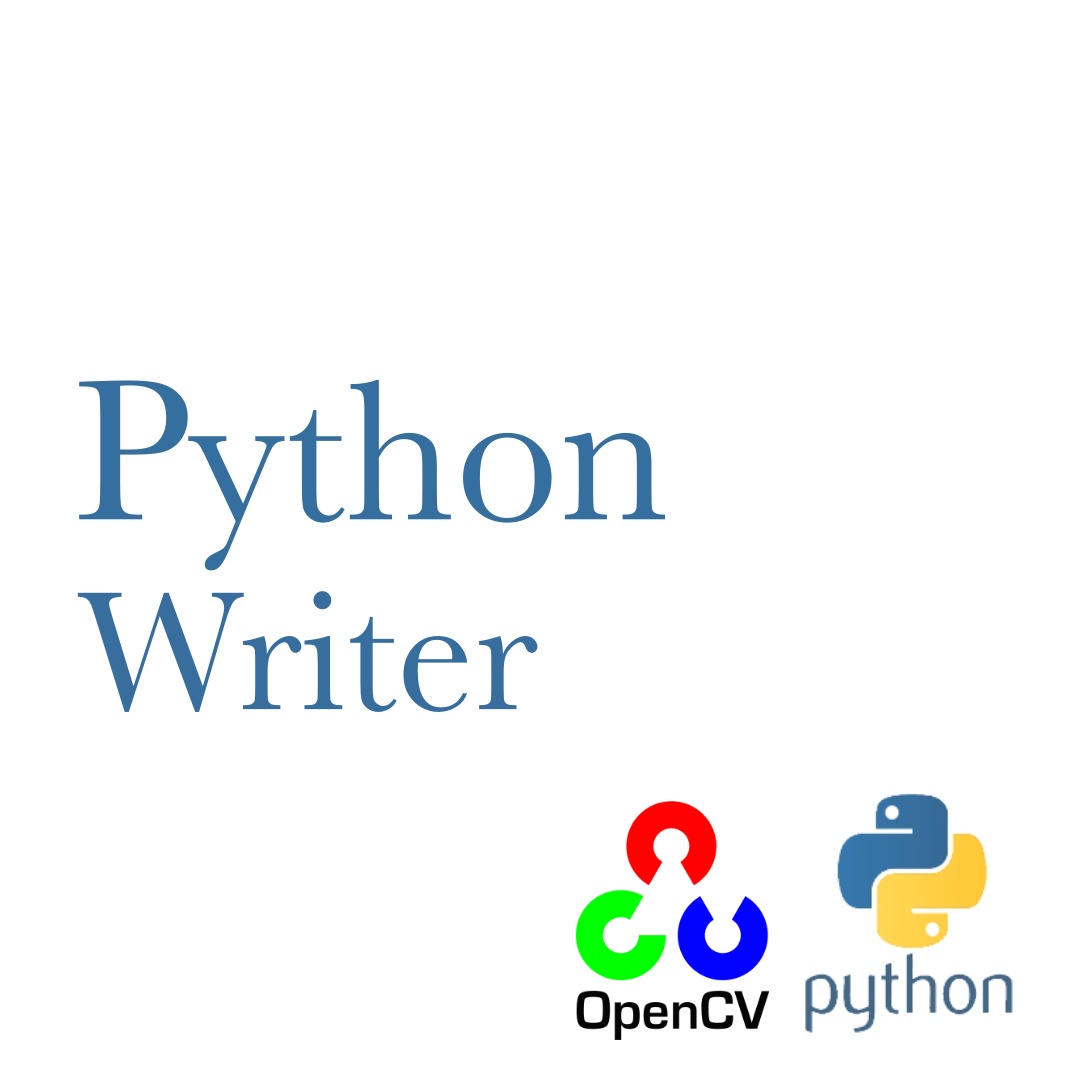
그리기¶
선 그리기¶
white 배경 생성 및 pt1-pt2(Red), pt1-pt3(Blue)를 잇는 선 그리기
cv2.line(배경, 좌표1, 좌표2, BGR value, line width) 으로 선 생성 가능
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pt1 = 0,0
pt2 = 500, 0
pt3 = 0, 500
cv2.line(img, pt1, pt2, (255,0,0), 5)
cv2.line(img, pt1, pt3, (0,0,255), 5)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
사각형 그리기¶
white 배경 생성 및 pt1-pt4로 사각형 그리기
cv2.rectangle(배경, 좌표1, 좌표2, BGR value, line width)으로 사각형 생성 가능
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pt1 = 0,0
pt4 = 400, 400
cv2.rectangle(img, pt1, pt4, (0,255,0), 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
선과 사각형 그리기¶
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pt1 = 0,0
pt2 = 500, 0
pt3 = 0, 500
pt4 = 400, 400
cv2.line(img, pt1, pt2, (255,0,0), 5)
cv2.line(img, pt1, pt3, (0,0,255), 5)
cv2.rectangle(img, pt1, pt4, (0,255,0), 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
clipline¶
white 배경 생성 및 pt1-pt4로 사각형 그리기
cv2.clipline(검충 하려는 직사각형 영역, 직선의 시작점, 직선의 종료점, retval, 사각형 안에 있는 선의 시작점, 사각형 안에 있는 선의 종료점)으로 사각형 생성 가능
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pt1 = 120, 50
pt2 = 300, 500
x1, x2, y1, y2 = 100, 400, 100, 400
cv2.rectangle(img, (x1, y1), (x2, y2), (0,0,255))
cv2.line(img, pt1, pgt2, (255, 0, 0), 2)
imgRect = (x1, y1, x2-x1, y2-y1)
cv2.clipLine(imRect, pt1, pt2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
circle¶
circle() 함수로 구현 가능
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
cx = img.shape[0]//2
cy = img.shape[1]//2
cv2.circle(img,(cx, cy), 200, (0,0,255))
cv2.circle(img,(cx, cy), 100, (0,0,255))
cv2.circle(img,(cx, cy), 10, (255,0,0))
cv2.circle(img,(cx, cy), 1, (0,0,1))
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
Ellipse¶
ellipse() 함수로 구현 가능
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
cx = img.shape[0]//2
cy = img.shape[1]//2
cv2.ellipse(img,(cx, cy), (90, 50), 50, 50, 360, (0,0,255))
cv2.ellipse(img,(cx, cy), (90, 50), -50, 50, 360, (255,0,0))
cv2.ellipse(img,(cx, cy), (90, 50), 50, -50, 360, (0,255,0))
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
Ellipse(box)¶
ellipse() 함수로 구현 가능
box option 이용
In [ ]:
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
center = img.shape[0]//2, img.shape[1]//2
size = 200, 100
box = (center, size, 0)
cv2.ellipse(img, box, (255, 0, 0), 5)
box = (center, size, 45)
cv2.ellipse(img, box, (0, 0, 255), 5)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
polylines()¶
여러개의 좌표를 지정하여 다각형을 구현합니다.
In [ ]:
import cv2
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pts1 = np.array([[100, 200], [200, 200], [200, 100], [100, 100]])
pts2 = np.array([[300, 200], [400, 200], [400, 100]]) # 3개의 포인트 지정
cv2.polylines(img, [pts1], True, (255,0,0), 2)
cv2.polylines(img, [pts2], True, (255,0,0), 2)
pts3 = np.array([[100, 400], [200, 400], [200, 300], [100, 300]])
cv2.polylines(img, [pts3], False, (0,0,255), 2)
cv2.imshow('polylines', img )
cv2.waitKey()
cv2.destroyAllWindows()
ellipse2Poly()¶
delta각의 의하여 연결되는 선들을 이용하여 원에 가까운 다각형을 그릴 수 있다. delta를 제외한 파라미터는 elipse와 동일하다.
delta(각도)값이 작아질 수 록 좌표가 많아진다.
In [ ]:
import cv2
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
ptCenter = img.shape[0]//2, img.shape[1]//2
size = 200, 100
cv2.ellipse(img, ptCenter, size, 0, 0, 360, (0,255,0))
pts1 = cv2.ellipse2Poly(ptCenter, size, 0, 0, 360, delta = 50)
cv2.polylines(img, [pts1], False, (255,0,0), 2)
cv2.ellipse(img, ptCenter, size, 90, 0, 360, (0,0,255))
pts2 = cv2.ellipse2Poly(ptCenter, size, 90, 0, 360, delta = 50)
cv2.polylines(img, [pts2], False, (0,255,0), 2)
cv2.imshow('polylines', img)
cv2.waitKey()
cv2.destroyAllWindows()
rotated rectangle¶
angle을 변경 다양한 각도로 사각형 생성
In [ ]:
import cv2
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
x, y, size, angle = 150, 250, 100, 45
rect = ((x,y),(size,size), angle)
box = cv2.boxPoints(rect).astype(np.int32)
print('box = ', box)
r, g, b = np.random.randint(256), np.random.randint(256), np.random.randint(256
cv2.polylines(img, [box], True, (r,g,b), 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
box = [[ 79 250]
[150 179]
[220 250]
[150 320]]
Utilize repeat statements¶
In [ ]:
import cv2
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
x, y, size = 150, 250, 100
for angle in range(0, 90, 10) :
rect = ((x,y),(size,size), angle)
box = cv2.boxPoints(rect).astype(np.int32)
r, g, b = np.random.randint(256), np.random.randint(256), np.random.randint(256)
cv2.polylines(img, [box], True, (r,g,b), 1)
print('box = ', box)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
box = [[ 92 209]
[190 192]
[207 290]
[109 307]]
In [ ]:
import cv2
import numpy as np
img = np.zeros(shape=(512, 512, 3), dtype=np.uint8) + 255
pts1 = np.array([[100, 200], [200, 200], [200, 100], [100, 100]])
pts2 = np.array([[300,200], [400,200], [400,100]])
cv2.fillConvexPoly(img, pts1, (255,0,0))
cv2.fillPoly(img, [pts2], (0,0,255))
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
fillPoly()으로 ploygon 구현¶
2개의 다각형 구현
In [ ]:
import cv2
import numpy as np
img1 = np.zeros(shape=(400, 400, 3), dtype=np.uint8)
img2 = np.zeros(shape=(400, 400, 3), dtype=np.uint8)
pt1 = np.array([[100, 100], [270, 110], [300, 330], [170, 170], [150, 250]], np.int32)
cv2.fillConvexPoly(img1, pt1, (255,0,0))
cv2.fillPoly(img2, [pt1], (0,0,255))
cv2.imshow('convex', img1)
cv2.imshow('poly', img2)
cv2.waitKey()
cv2.destroyAllWindows()
반응형
'Python' 카테고리의 다른 글
[Python] openCV : Event (0) | 2022.11.10 |
---|---|
[Python] openCV : Text (0) | 2022.11.10 |
[Python] openCV : Image display (0) | 2022.11.10 |
[Python] Open CV : 개요 (0) | 2022.11.10 |
[Python] Regression (0) | 2022.11.10 |