반응형
문제
- 추가 버튼을 누르면 테이블 row가 추가되고 초기화 버튼을 누르면 초기화 되는 코드를 작성하시오.
- 과일명은 '사과', '배', '딸기', '바나나', '귤' 중 랜덤 값
- 수량은 1~10 사이 랜덤 값
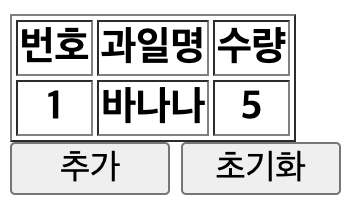
해법
1. body에 table과 button을 생성한다.
<body>
<table border="1px">
<tr>
<th>번호</th><th>과일명</th><th>수량</th>
</tr>
</table>
<button id="id_addBtn" style="width : 80px">추가</button> <button id="id_delBtn" style="width: 80px;">초기화</button>
</body>
2. 순차적으로 번호를 뽑는 함수, 과일명을 랜덤으로 리턴하는 함수, 수량 값을 랜덤으로 리턴하는 함수를 생성한다.
//순차적 번호 리턴 함수
let _num = 0;
const num = function (){
_num = _num + 1;
return _num;
}
//과일명 랜덤 리턴 함수
const frtNme = function(){
const frt = ['사과', '배', '딸기', '바나나', '귤']
let index = Math.floor(Math.random() * 5)
return frt[index];
}
//수량 값 랜덤 리턴 함수
const ranNum = function(){
const rNum = Math.floor((Math.random() * 10) + 1)
return rNum;
}
3. 추가, 초기화 버튼에 이벤트를 생성하여 출력한다.
document.addEventListener('DOMContentLoaded', () => {
//통합 테이블 생성
const tableTag = document.querySelector('table')
//추가, 초화 버튼 생성
const addBtnTag = document.querySelector('#id_addBtn')
const delBtnTag = document.querySelector('#id_delBtn')
//추가 버튼 이벤트 + 기능
addBtnTag.addEventListener('click', () => {
const trTag = document.createElement('tr')
const thNTag = document.createElement('th')
const thFrtTag = document.createElement('th')
const thNumFrtTag = document.createElement('th')
//상속
trTag.append(thNTag, thFrtTag, thNumFrtTag)
tableTag.appendChild(trTag)
//출력
thNTag.textContent = `${num()}`
thFrtTag.textContent = `${frtNme()}`
thNumFrtTag.textContent = `${ranNum()}`
//초기화 버튼 이벤트 + 기능
delBtnTag.addEventListener('click', () => {
tableTag.removeChild(trTag)
_num = 0;
})
})
})
Code
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script>
//추가 버튼을 누르면 테이블row가 추가되고 초기화버튼을 누르면 초기화 되는 코드를 작성하시오.
//과일명은 사과, 배, 딸기, 바나나, 귤 중 랜덤 1
//수량은 1~10 사이 랜덤값
document.addEventListener('DOMContentLoaded', () => {
//통합 테이블 생성
const tableTag = document.querySelector('table')
//추가, 초화 버튼 생성
const addBtnTag = document.querySelector('#id_addBtn')
const delBtnTag = document.querySelector('#id_delBtn')
//추가 버튼 이벤트 + 기능
addBtnTag.addEventListener('click', () => {
const trTag = document.createElement('tr')
const thNTag = document.createElement('th')
const thFrtTag = document.createElement('th')
const thNumFrtTag = document.createElement('th')
//상속
trTag.append(thNTag, thFrtTag, thNumFrtTag)
tableTag.appendChild(trTag)
//출력
thNTag.textContent = `${num()}`
thFrtTag.textContent = `${frtNme()}`
thNumFrtTag.textContent = `${ranNum()}`
//초기화 버튼 이벤트 + 기능
delBtnTag.addEventListener('click', () => {
tableTag.removeChild(trTag)
_num = 0;
})
})
})
//순차적 번호 리턴 함수
let _num = 0;
const num = function (){
_num = _num + 1;
return _num;
}
//과일명 랜덤 리턴 함수
const frtNme = function(){
const frt = ['사과', '배', '딸기', '바나나', '귤']
let index = Math.floor(Math.random() * 5)
return frt[index];
}
//수량 값 랜덤 리턴 함수
const ranNum = function(){
const rNum = Math.floor((Math.random() * 10) + 1)
return rNum;
}
</script>
</head>
<body>
<table border="1px">
<tr>
<th>번호</th><th>과일명</th><th>수량</th>
</tr>
</table>
<button id="id_addBtn" style="width : 80px">추가</button> <button id="id_delBtn" style="width: 80px;">초기화</button>
</body>
</html>
번호 | 과일명 | 수량 |
---|
반응형
'JavaScript' 카테고리의 다른 글
[JavaScript] UI 설계 예제 (0) | 2022.05.04 |
---|---|
[JavaScript] checkbox 전체 설정하기 (0) | 2022.05.02 |
[JavaScript] 주사위의 값 구하기 (0) | 2022.05.01 |
[JavaScript] 원주율에 따른 원의 넓이, 둘레 구하기 (예제) (0) | 2022.04.27 |
[JavaScript] Event +예제 (0) | 2022.04.26 |