반응형
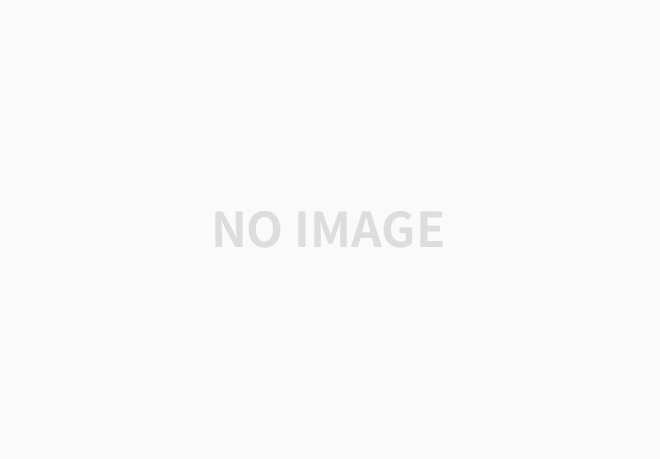
new.target
new.target 속성(property)은 함수 또는 생성자가 new 연산자를 사용하여 호출됐는지를 감지할 수 있습니다.
new 연산자로 인스턴스화된 생성자 및 함수에서, new.target은 생성자 또는 함수 참조를 반환합니다.
일반 함수 호출에서는, new.target은 undefined입니다.
구문
new.target
예시
<body>
<script>
const func = function()
{
if(!new.target) //생성자가 아닌것
{
return "This is not Constructor."
}
else
{
return "This is Constructor."
}
}
const a = func()
console.log(a)
//This is not Constructor.
</script>
</body>
예제
일반 함수와 생성자를 이용해 두개의 수를 더하는 함수를 만드시오.
<body>
<script>
const add = function (_a, _b)
{
if (new.target) //생성자인 경우
{
this.a = _a
this.b = _b
//a와b의 getter, setter
this.getA = function()
{
return this.a
}
this.getB = function()
{
return this.b
}
this.setA = function(_a)
{
this.a = _a
}
this.setB = function(_b)
{
this.a = _b
}
//더하는 함수
this.getAdd = function()
{
return this.a + this.b
}
}
else
{
return _a + _b
}
}
const a = add(10, 30)
const b = new add(10, 9)
console.log(a)
console.log(b.getAdd())
//40
//19
</script>
</body>
예제2
아래와 같은 코드는 obj가 출력되는 문제가 있다.
아래의 코드를 생성자로 출력할시와 일반 함수로 출력하는 함수를 구하시오.
<body>
<script>
const add = function(a, b)
{
return a + b;
}
const obj = new add(1, 2)
console.log(obj);
//obj
</script>
</body>
답)
<body>
<script>
const add = function(_a, _b)
{
if (new.target){
this.a = _a
this.b = _b
this.getAdd = function(){
return this.a + this.b
}
}
else {
return _a + _b;
}
}
const obj = new add(10, 20)
const obj2 = add(1, 2)
console.log(obj.getAdd());
console.log(obj2)
//30
//3
</script>
</body>
반응형
'JavaScript' 카테고리의 다른 글
[JavaScript] Class(클래스), private(접근제어) (0) | 2022.04.20 |
---|---|
[JavaScript] Constructor 예제 (2) | 2022.04.19 |
[JavaScript] Prototype (0) | 2022.04.19 |
[JavaScript] Closure(클로저) 예제 (0) | 2022.04.18 |
[JavaScript] 객체지향, Constructor(생성자), new (0) | 2022.04.18 |